The answer is for ADO.
1) how can i add records to an existing table of a database SQL in "batch" mode (not interactively with browse and similar)?
You can do it in two ways.
(a) Directly using SQL.
(b) Opening recordset in batchmode.
(a) Directly using SQL.
SQL syntax is different for different RDMS like MYSql, MsSql, Oracle, etc. It is also extremely cumborsome to prepare SQL statements. You might have seen lots postings as to how to prepare the sql statement for insert, update, etc.
If you use FWH provided commands and functions there is no scope for any confusion or errors. These commands/functions prepare the sql statements using the syntax applicable to the RDBMS connected by using FW_OpenAdoConnection(), be it MySql, MsSql, Oracle, etc.
This sample demonstrates inserting bulk records using SQL statement. For the purpose of this example, we are using the Demo server of FWH. You may use connecting to your own server later.
Please copy the program to fwh\samples folder and build the exe using buildh.bat or buildx.bat
- Code: Select all Expand view RUN
#include "fivewin.ch"
#include "adodef.ch"
function Main()
local oCn, cSql, aData
local oRs, n
oCn := FW_DemoDB( "ADO" ) // You may use your own connection
// CREATE A TABLE FOR TEST
TRY
oCn:Execute( "DROP TABLE instest" )
CATCH
END
FWAdoCreateTable( "instest", { ;
{ "name", "C", 10, 0 }, ;
{ "amount","N", 10, 2 }, ;
{ "date", "D", 8, 0 } }, ;
oCn )
// SAMPLE DATA TO BE INSERTED.
aData := { ;
{ "David", 2000, Date() - 2000 }, ;
{ "John", 3000, Date() - 1000 }, ;
{ "James", 5000, Date() - 500 } }
// This command prepares the actual SQL to be used for insertion
cSql := SQL INSERT INTO instest ( name,amount,date ) ARRAY aData
MEMOEDIT( cSql ) // View the SQL for your information.
oCn:Execute( cSql ) // insert all records in a single batch
? "Inserted"
// Check if the data is inserted
oRs := FW_OpenRecordSet( oCn, "instest" )
XBROWSER oRs FASTEDIT
oRs:Close()
oCn:Close()
return nil
b) Using RecordSet opened in Batch mode
- Code: Select all Expand view RUN
#include "fivewin.ch"
#include "adodef.ch"
function Main()
local oCn, cSql, aData
local oRs, n
oCn := FW_DemoDB( "ADO" ) // You may use your own connection
// CREATE A TABLE FOR TEST
TRY
oCn:Execute( "DROP TABLE instest" )
CATCH
END
FWAdoCreateTable( "instest", { ;
{ "name", "C", 10, 0 }, ;
{ "amount","N", 10, 2 }, ;
{ "date", "D", 8, 0 } }, ;
oCn )
// SAMPLE DATA TO BE INSERTED.
aData := { ;
{ "David", 2000, Date() - 2000 }, ;
{ "John", 3000, Date() - 1000 }, ;
{ "James", 5000, Date() - 500 } }
? "Start"
oRs := FW_OpenRecordSet( oCn, "instest", adLockBatchOptimistic )
for n := 1 to Len( aData )
oRs:AddNew( { "name", "amount", "date" }, aData[ n ] )
next
oRs:UpdateBatch() // All records are inserted on the server in one batch
oRs:Close()
? "Inserted"
// Check if the data is inserted
oRs := FW_OpenRecordSet( oCn, "instest" )
XBROWSER oRs FASTEDIT
oRs:Close()
oCn:Close()
return nil
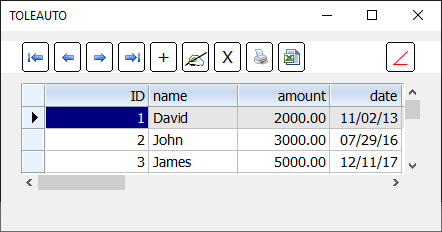