It is necessary that the remote server already has identical tables with identical structures and records with the same names. This can be setup initially by using
- Code: Select all Expand view
oCn:CopyTableToServer( cTable, oRemoteServer )
Using the replication feature:
Open connections to main server and also replication server.
- Code: Select all Expand view
oCn := maria_connect( <mainserver>, .... )
oRemote := maria_connect( <remote server>, ... )
Open the rowset for the table to edit in the usual way.
- Code: Select all Expand view
oRs := oCn:RowSet( <mytable> )
The only additional work we need to do for the replication is to assign oRs:oCn2 with the connection to remote server
- Code: Select all Expand view
oRs:oCn2 := oRemote
This is enough.
All changes made to <mytable> through the methods of RowSet are automatically written simultaneously to the same table on the remote server also.
Conditions:
Do not change the table with your own SQL statements.
Do not directly modify the replicated tables on the remote server.
\fwh\samples\mariarpl.prg
- Code: Select all Expand view
- #include "fivewin.ch"
function Main()
local oMain := FW_DemoDB()
local oRepl := FW_DemoDB( 6 )
local oRsMain, oRsRepl
local oDlg, oFont, oBold, oBrwMain, oBrwRepl
SetGetColorFocus()
oRsMain := oMain:RowSet( "states" )
oRsMain:oCn2 := oRepl
oRsRepl := oRepl:RowSet( "states", , .t. ) // readonly
DEFINE FONT oFont NAME "TAHOMA" SIZE 0,-14
oBold := oFont:Bold()
DEFINE DIALOG oDlg SIZE 900,500 PIXEL TRUEPIXEL FONT oFont ;
TITLE "FWH 18.11 : TABLE REPLICATION"
@ 20, 20 XBROWSE oBrwMain SIZE 425,-20 PIXEL OF oDlg ;
DATASOURCE oRsMain ;
AUTOCOLS CELL LINES NOBORDER
@ 20,455 XBROWSE oBrwRepl SIZE -20,-20 PIXEL OF oDlg ;
DATASOURCE oRsRepl ;
AUTOCOLS CELL LINES NOBORDER
WITH OBJECT oBrwMain
:SetGroupHeader( "MAIN SERVER", 1, 3, oBold )
:nEditTypes := EDIT_GET
:bOnChanges := { || oRsRepl:ReSync(), oBrwRepl:RefreshCurrent() }
:lColChangeNotify := .t.
:bChange := { || oBrwRepl:BookMark := oBrwMain:BookMark, ;
oBrwRepl:nRowSel := oBrwMain:nRowSel, ;
oBrwRepl:nColSel := oBrwMain:nColSel, ;
oBrwRepl:Refresh() }
//
:CreateFromCode()
END
WITH OBJECT oBrwRepl
:SetGroupHeader( "REPLICATION SERVER", 1, 3, oBold )
:bClrSel := { || { CLR_WHITE, CLR_GREEN } }
//
:CreateFromCode()
END
ACTIVATE DIALOG oDlg CENTERED
RELEASE FONT oFont, oBold
oRsMain := nil
oRsRepl := nil
oMain:Close()
oRepl:Close()
return nil
Note: It is not necessary to open the table on the remote server. This is opened in the above sample to demonstrate simultaneous changes to the remote table.
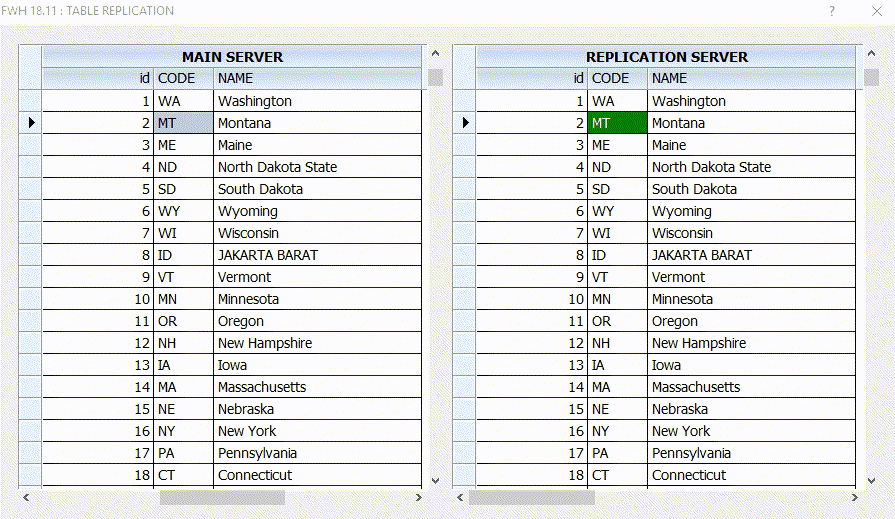