Why is it so important ? https://youtu.be/p9IsfqNewmE
1. Download and install OpenCV – 4.5.4 for Windows from https://opencv.org/releases/
2. Install it at c:\opencv
3. Copy c:\opencv\build\x64\vc15\bin\opencv_world454.dll to the folder where you are going to build this test
This tutorial requires FWH 64 bits and Microsoft Visual Studio Community.
Edited: Examples posted here don't require FWH 64 at all.
cv1.prg
Code: Select all | Expand
#include "FiveWin.ch"
function Main()
Test()
return nil
#pragma BEGINDUMP
#include <hbapi.h>
#include <opencv.hpp>
using namespace cv;
HB_FUNC( TEST )
{
cv::Mat img = imread( "007.jpg" );
namedWindow( "image", WINDOW_AUTOSIZE );
imshow( "image", img );
waitKey( 0 );
}
#pragma ENDDUMP
go64.bat
Code: Select all | Expand
@set oldpath=%Path%
@set oldinclude=%INCLUDE%
call "%ProgramFiles(x86)%\Microsoft Visual Studio\2019\Community\VC\Auxiliary\Build\vcvarsall.bat" x86_amd64
c:\harbour\bin\win\msvc64\hbmk2 cv1.hbp -comp=msvc64
@set Path=%oldpath%
@set INCLUDE=%oldinclude%
cv1.hbp
Code: Select all | Expand
cv1.prg
-cflag=-TP
-Ic:\fwh64\include
-Ic:\opencv\build\include
-Ic:\opencv\build\include\opencv2
-Lc:\fwh64\lib
-lfiveh64
-lfivehc64
-lgdiplus
-lversion
-lOleDlg
-lopencv_world454
-gui
xhb.hbc
hbct.hbc
hbwin.hbc
hbmzip.hbc
hbziparc.hbc
hbfoxpro.hbc
opencvlib.hbp
opencvlib.hbp
Code: Select all | Expand
-hbimplib opencv_world454.dll
Save this image as 007.jpg
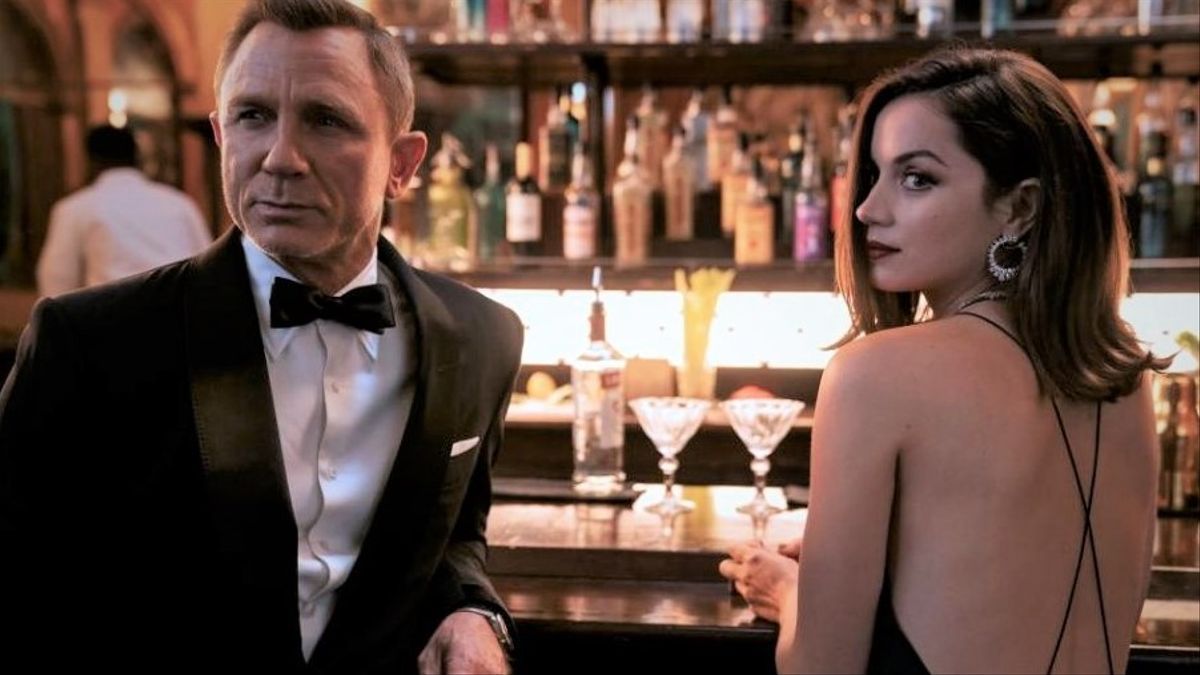
When you run cv1.exe you get this:
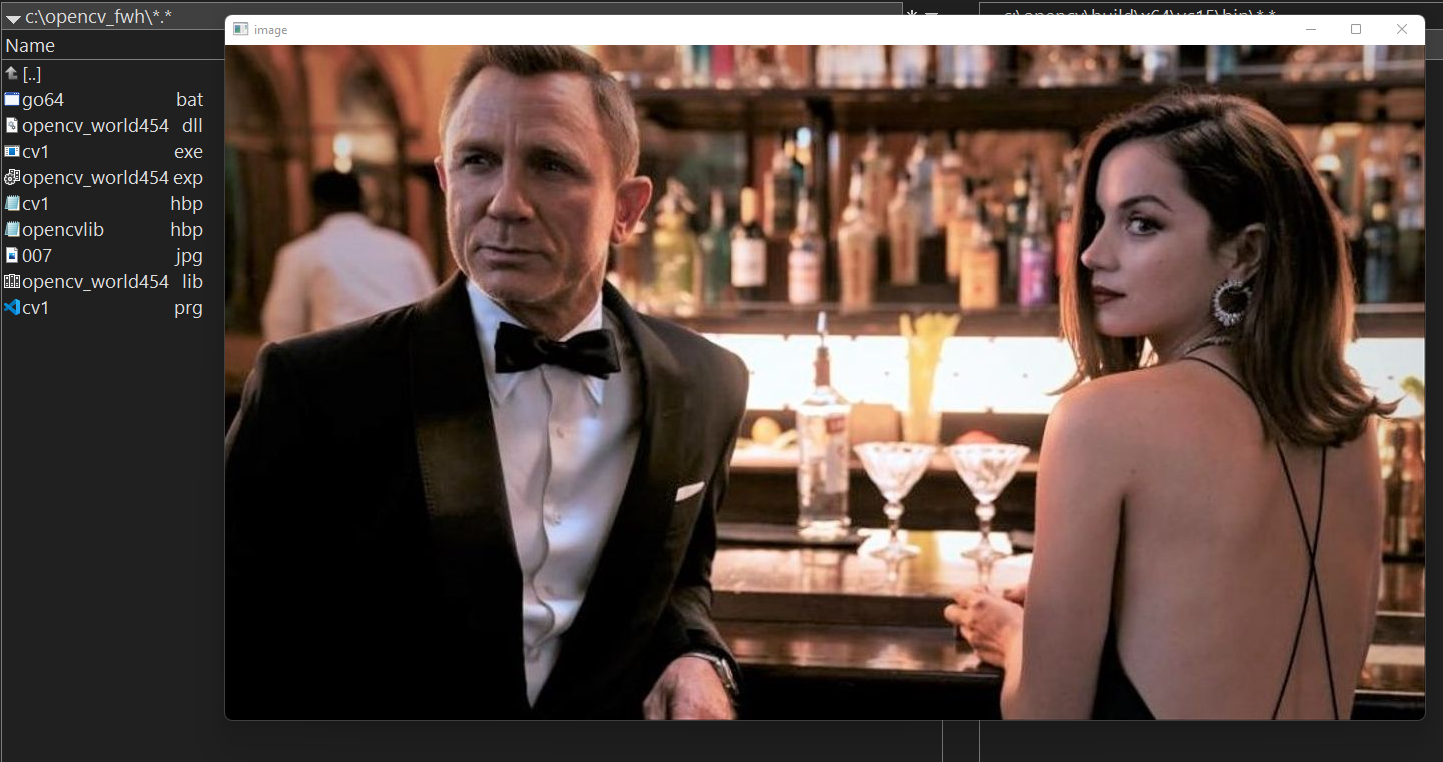