webviewlogin.prg
- Code: Select all Expand view RUN
- // Please install https://developer.microsoft.com/en-us/m ... /webview2/ x86 version before using it
#include "FiveWin.ch"
function Main()
local oWebView := TWebView():New()
oWebView:SetHtml( Html() )
oWebView:SetTitle( "Please identify with your credentials" )
oWebView:SetSize( 1200, 800 )
oWebView:SetUserAgent( "Mozilla/5.0 (Linux; Android 6.0; Nexus 5 Build/MRA58N) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/103.0.5060.53 Mobile Safari/537.36" )
oWebView:Bind( "SendToFWH" )
oWebView:bOnBind = { | cJson, cCalls | Login( cJson, cCalls, oWebView ) }
sleep( 300 )
oWebView:Run()
oWebView:Destroy()
return nil
function Login( cJson, cCalls, oWebView )
local hData
hb_jsonDecode( cJson, @hData )
if hData[ 1 ][ "username" ] != "Antonio" .or. hData[ 1 ][ "password" ] != "1234"
oWebView:Return( cCalls, 0, "{ 'result': 'incorrect values' }" )
else
oWebView:Return( cCalls, 0, "{ 'result': 'correct!' }" )
endif
return nil
function Html()
local cHtml
TEXT INTO cHtml
<!DOCTYPE html>
<html>
<head>
<title>Identify</title>
<style>
body {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #F2F2F2;
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
.container {
max-width: 400px;
margin: 0 auto;
padding: 40px;
background-color: #FFFFFF;
border-radius: 5px;
display: flex;
flex-direction: column;
align-items: center;
box-shadow: 0px 0px 44px rgba(0, 0, 0, 0.7) !important;
overflow:none !important;
}
.logo {
margin-bottom: 40px;
display: flex;
align-items: center;
}
.logo img {
max-width: 100%;
height: auto;
}
.btn {
display: inline-block;
padding: 12px 24px;
background-color: #4CAF50;
color: #FFFFFF;
font-size: 16px;
text-decoration: none;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.btn:hover {
background-color: #45A049;
}
.form-group {
margin-bottom: 20px;
}
.form-group label {
display: block;
font-size: 16px;
font-weight: bold;
margin-bottom: 5px;
color: #333333;
}
.form-group input {
width: 100%;
padding: 12px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #CCCCCC;
}
.btn {
display: inline-block;
padding: 12px 24px;
background-color: #4CAF50;
color: #FFFFFF;
font-size: 16px;
text-decoration: none;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.btn:hover {
background-color: #45A049;
}
body {
background-color: #3498db;
}
.btn {
background-color: #2980b9;
}
.btn:hover {
background-color: #1a5276;
.logo {
margin-bottom: 40px;
}
.logo img {
max-width: 100%;
height: auto;
}
}
.myinput {
width: auto !important;
}
.mybtn {
text-align:center;
}
</style>
</head>
<body>
<div class="container">
<div class="logo">
<img src="https://fivetechsupport.com/forums/styles/prosilver/imageset/site_logo.gif" alt="Logo">
</div>
<form id="login-form" action="#" method="POST">
<div class="form-group">
<label for="username">Username:</label>
<input type="text" id="username" name="username" class="myinput" required>
</div>
<div class="form-group">
<label for="password">Password:</label>
<input type="password" id="password" name="password" class="myinput" required>
</div>
<div class="form-group mybtn" >
<button type="submit" class="btn">Iniciar sesión</button>
</div>
</form>
</div>
<script>
document.getElementById('login-form').addEventListener('submit', function(event) {
event.preventDefault();
var username = document.getElementById('username').value;
var password = document.getElementById('password').value;
var data = {
username: username,
password: password
};
var s = SendToFWH(data).then( s => { alert(s.result); } );
});
</script>
</body>
</html>
ENDTEXT
return cHtml
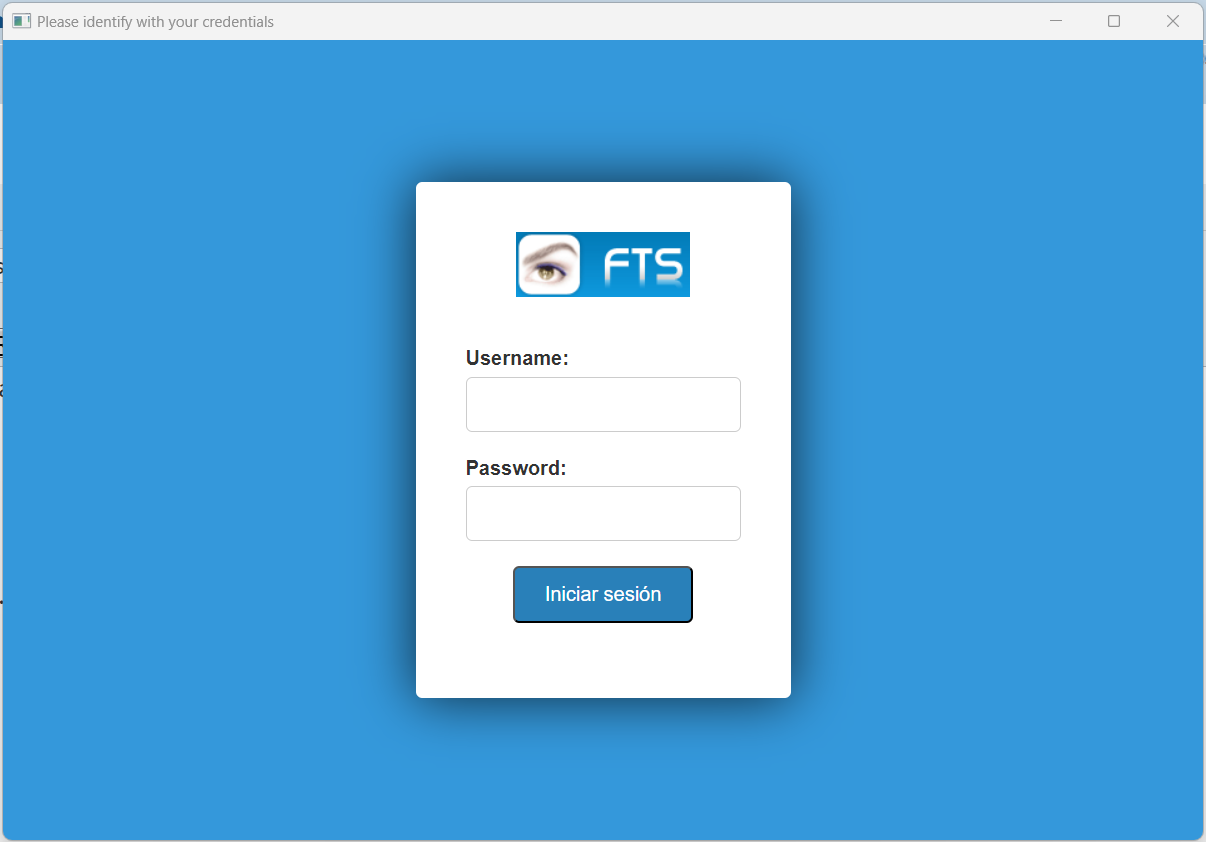