Need Help guide for my program.
-
- Posts: 85
- Joined: Wed Nov 19, 2014 1:04 pm
- Contact:
Need Help guide for my program.
Good morning
For Jewellery business. We may received Payments (Cash Rs, Old Gold, Old Silver) against
1. Order (Amts must display below order after making invoice for that order amts paid should display below the invoice)
2. Advance payment withour order.
3. Against Bill. (Payment amt must display below the Bill).
How-to accomplish it?
Regards
Yunus
For Jewellery business. We may received Payments (Cash Rs, Old Gold, Old Silver) against
1. Order (Amts must display below order after making invoice for that order amts paid should display below the invoice)
2. Advance payment withour order.
3. Against Bill. (Payment amt must display below the Bill).
How-to accomplish it?
Regards
Yunus
Last edited by dagiayunus on Tue Jul 30, 2024 5:13 pm, edited 1 time in total.
Regards
Yunus
FWH 21.02
Yunus
FWH 21.02
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
Hello Yunus,
Isn't this simply a deposit management system?
You convert the old gold and silver directly into a monetary value, don't you?
Wouldn't this be treated similarly to advance payments in a hotel?
Best regards,
Otto
PS: Are you the namesake and programmer of yunos.prg in the FIVEWIN samples?
Isn't this simply a deposit management system?
You convert the old gold and silver directly into a monetary value, don't you?
Wouldn't this be treated similarly to advance payments in a hotel?
Best regards,
Otto
PS: Are you the namesake and programmer of yunos.prg in the FIVEWIN samples?
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
-
- Posts: 85
- Joined: Wed Nov 19, 2014 1:04 pm
- Contact:
Re: Need Help giide for my program
Dear Mr.Otto
Yes it is me. That program was created by Mr.Antonio and Mr.Rao on my request to learn Fivewin.
Regards
Yunus
Yes it is me. That program was created by Mr.Antonio and Mr.Rao on my request to learn Fivewin.
Regards
Yunus
Regards
Yunus
FWH 21.02
Yunus
FWH 21.02
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
Hello Yunus,
start with a ChatGPT prompt.
Best regards,
Otto
start with a ChatGPT prompt.
Best regards,
Otto
To modify your program so that it works similarly for deposits as it does for invoices, you need to ensure that all the relevant functions and structures for deposits mirror those of invoices.
Here's how you can adapt the Invoices() function to create a Deposits() function and ensure that it operates correctly:
Copy and Adapt Invoices Function: First, copy the Invoices function and rename it to Deposits. Replace all instances of Invoices with Deposits and make sure to update all relevant database names and column headers to match the structure of your deposits data.
Database Setup: Ensure you have a deposits.dbf database file similar to the invoices.dbf and that it has the necessary fields. Also, ensure that your OpenDataBases() function initializes the deposits.dbf correctly.
Menu and Button Actions: Make sure that your menu and button actions call the new Deposits() function instead of the Invoices() function when the user selects deposits.
Below is the adapted Deposits function based on the Invoices function:
xbase
Code kopieren
static function Deposits()
local oBrw, oChild, cClrBack, cCol
local oBar, oMsgBar, oMsgDeleted
if oWndClients == nil
Clients()
endif
if oWndItems == nil
Items()
endif
if oWndDeposits == nil
DEFINE WINDOW oWndDeposits MDICHILD OF oWndMain TITLE "Deposits"
@ 60, 0 XBROWSE oBrw SIZE 0,200 PIXEL OF oWndDeposits LINES AUTOSORT ;
AUTOCOLS DATASOURCE Alias() NOBORDER FOOTERS
oBar := BrwBtnBar( @oBrw, oWndDeposits )
DEFINE BUTTON OF oBar PROMPT "Print" RESOURCE "report" ;
ACTION (oBrw:bClrStds := {||{ CLR_WHITE,CLR_BLACK }}, oBrw:refresh())
DEFINE BUTTON OF oBar PROMPT "Print" RESOURCE "report" ;
ACTION ViewDeposit( oBrw )
DEFINE BUTTON OF oBar PROMPT "HTML Report" RESOURCE "report" ;
ACTION ViewDepositHTML( oBrw )
DEFINE BUTTON OF oBar PROMPT "Close" RESOURCE "exit" ;
ACTION oWndDeposits:End()
DEFINE MSGBAR oMsgBar OF oWndDeposits 2007
BrwColors( oBrw )
BrwRecSel( oBrw, "RECNO" )
for each cCol in { "Amount", "Tax", "Total" }
oBrw:oCol( cCol ):nFooterType := AGGR_SUM
next
oBrw:bLDblClick = { || oBrw:EditSource(,, .T.) }
oBrw:bEdit = { | oRec | EditDeposit( oRec ) }
oBrw:MakeTotals()
oBrw:CreateFromCode()
oBrw:SetFocus()
oWndDeposits:oControl = oBrw
@ oBar:nHeight + 200,0 XBROWSE oChild SIZE 0,-oMsgBar:nHeight PIXEL OF oWndDeposits ;
DATASOURCE "depItems" ;
COLUMNS "ItemCode", "ItemName", "Quantity", "Unit", "Price", ;
"ROUND(QUANTITY*PRICE,0)", "DISCOUNT","ROUND(QUANTITY*PRICE,0)-DISCOUNT" ;
HEADERS "ItmCode", nil, nil, nil, nil, "Amount", "Discount", "Net Amount" ;
LINES NOBORDER FOOTERS
BrwColors( oChild )
BrwRecSel( oChild, "KEY" )
for each cCol in { "Amount", "Discount", "Net Amount" }
WITH OBJECT oChild:oCol( cCol )
:nFooterType := AGGR_SUM
END
next
oChild:MakeTotals()
oChild:CreateFromCode()
oBrw:bChange := { || oChild:Refresh(), oChild:MakeTotals(), oChild:GoTop() }
oWndDeposits:bResized := < ||
local oRect := oWndDeposits:GetCliRect()
oBrw:nHeight := ( oRect:nHeight - oBar:nHeight - oMsgBar:nHeight ) * 0.6
oChild:nTop := oBrw:nTop + oBrw:nHeight
return nil
>
oWndDeposits:bPostEnd := { || oWndDeposits := nil }
if cRequest = "online"
oBrw:seek("DEP003")
ACTIVATE WINDOW oWndDeposits MAXIMIZED ;
ON INIT ( ViewDeposit( oBrw ), oWndClients:END() );
VALID ( oWndClients := nil, .T. )
else
ACTIVATE WINDOW oWndDeposits MAXIMIZED
endif
else
oWndDeposits:SetFocus()
endif
return nil
In this function, make sure that all the database references, field names, and other specifics match your deposits setup.
Additional Steps:
Create and Index Deposits Database: Ensure you have a deposits.dbf file with the appropriate fields and indices similar to invoices.dbf.
Adapt Editing Functions: Similar to EditInvoice(), create an EditDeposit() function if the editing structure of deposits differs from invoices.
Menu and Button Integration: Ensure your menu and button actions call the Deposits() function appropriately. For example:
xbase
Code kopieren
DEFINE BUTTON OF oBar PROMPT "Deposit" RESOURCE "code" ;
ACTION Deposits()
This will ensure that your application handles deposits in a similar manner to invoices.
Last edited by Otto on Fri Jul 19, 2024 7:20 am, edited 1 time in total.
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
... as a next step ask ChatGPT how to make the link between invoice and deposit
To link invoices and deposits in your application, you need to establish a relationship between these two entities. This can be done by adding a reference field in the deposits database that links each deposit to its corresponding invoice. Here’s how you can implement this:
1. **Add a Reference Field in Deposits Database**: Add a field in the `deposits.dbf` file to store the invoice number that the deposit is related to. This can be called `INVNUM`.
2. **Modify Deposits Database Structure**: Ensure your `deposits.dbf` file includes the `INVNUM` field. Here’s how you can create or modify the deposits database structure:
```xbase
if !File( "deposits.dbf" )
DbCreate( "deposits.dbf",;
{ { "DEPONUM", "C", 10, 0 }, ;
{ "DATE", "D", 8, 0 }, ;
{ "CODE", "C", 10, 0 }, ; // Client Code
{ "CLIENT", "C", 40, 0 }, ; // Client Name
{ "ADDRESS", "M", 10, 0 }, ; // Client Address
{ "INVNUM", "C", 10, 0 }, ; // Invoice Number
{ "DETAILS", "C", 30, 0 }, ; // Deposit Details
{ "AMOUNT", "N", 9, 0 } }, "DBFCDX", .T., "DB" )
INDEX ON DEPONUM TAG DEPONUM
CLOSE DB
endif
```
3. **Modify `Deposits` Function**: Update the `Deposits()` function to include the `INVNUM` field in the XBROWSE and other relevant parts. Here’s an updated version of the `Deposits()` function to show the `INVNUM` field:
```xbase
static function Deposits()
local oBrw, oChild, cClrBack, cCol
local oBar, oMsgBar, oMsgDeleted
if oWndClients == nil
Clients()
endif
if oWndItems == nil
Items()
endif
if oWndDeposits == nil
DEFINE WINDOW oWndDeposits MDICHILD OF oWndMain TITLE "Deposits"
@ 60, 0 XBROWSE oBrw SIZE 0,200 PIXEL OF oWndDeposits LINES AUTOSORT ;
AUTOCOLS DATASOURCE Alias() NOBORDER FOOTERS
oBar := BrwBtnBar( @oBrw, oWndDeposits )
DEFINE BUTTON OF oBar PROMPT "Print" RESOURCE "report" ;
ACTION (oBrw:bClrStds := {||{ CLR_WHITE,CLR_BLACK }}, oBrw:refresh())
DEFINE BUTTON OF oBar PROMPT "Print" RESOURCE "report" ;
ACTION ViewDeposit( oBrw )
DEFINE BUTTON OF oBar PROMPT "HTML Report" RESOURCE "report" ;
ACTION ViewDepositHTML( oBrw )
DEFINE BUTTON OF oBar PROMPT "Close" RESOURCE "exit" ;
ACTION oWndDeposits:End()
DEFINE MSGBAR oMsgBar OF oWndDeposits 2007
BrwColors( oBrw )
BrwRecSel( oBrw, "RECNO" )
for each cCol in { "Amount", "Tax", "Total" }
oBrw:oCol( cCol ):nFooterType := AGGR_SUM
next
oBrw:bLDblClick = { || oBrw:EditSource(,, .T.) }
oBrw:bEdit = { | oRec | EditDeposit( oRec ) }
oBrw:MakeTotals()
oBrw:CreateFromCode()
oBrw:SetFocus()
oWndDeposits:oControl = oBrw
@ oBar:nHeight + 200,0 XBROWSE oChild SIZE 0,-oMsgBar:nHeight PIXEL OF oWndDeposits ;
DATASOURCE "depItems" ;
COLUMNS "ItemCode", "ItemName", "Quantity", "Unit", "Price", ;
"ROUND(QUANTITY*PRICE,0)", "DISCOUNT","ROUND(QUANTITY*PRICE,0)-DISCOUNT" ;
HEADERS "ItmCode", nil, nil, nil, nil, "Amount", "Discount", "Net Amount" ;
LINES NOBORDER FOOTERS
BrwColors( oChild )
BrwRecSel( oChild, "KEY" )
for each cCol in { "Amount", "Discount", "Net Amount" }
WITH OBJECT oChild:oCol( cCol )
:nFooterType := AGGR_SUM
END
next
oChild:MakeTotals()
oChild:CreateFromCode()
oBrw:bChange := { || oChild:Refresh(), oChild:MakeTotals(), oChild:GoTop() }
oWndDeposits:bResized := < ||
local oRect := oWndDeposits:GetCliRect()
oBrw:nHeight := ( oRect:nHeight - oBar:nHeight - oMsgBar:nHeight ) * 0.6
oChild:nTop := oBrw:nTop + oBrw:nHeight
return nil
>
oWndDeposits:bPostEnd := { || oWndDeposits := nil }
if cRequest = "online"
oBrw:seek("DEP003")
ACTIVATE WINDOW oWndDeposits MAXIMIZED ;
ON INIT ( ViewDeposit( oBrw ), oWndClients:END() );
VALID ( oWndClients := nil, .T. )
else
ACTIVATE WINDOW oWndDeposits MAXIMIZED
endif
else
oWndDeposits:SetFocus()
endif
return nil
```
4. **Modify `EditDeposit` Function**: Ensure that the `EditDeposit` function allows the user to link a deposit to an invoice by setting the `INVNUM` field.
```xbase
static function EditDeposit( oRec )
local lNew := ( oRec:RecNo == 0 )
local oDlg, oBrush, oFont, oBold, oLarge
local oBrw, cCol, bInit, oBtn
local aItems
local oGetClient, cClient, bCliInit
local nHt := Int( ScreenHeight() * 0.8 )
local nWd := 1100
local lSave := .f.
if lNew
oRec:Date := Date()
aItems := { AClone( aBlankItem ) }
else
aItems := IIT->( FW_DbfToArray( cItemFlds, { || IIT->INVNUM == oRec:InvNum } ) )
endif
DEFINE BRUSH oBrush RESOURCE "PAPER"
DEFINE FONT oLarge NAME "VERDANA" SIZE 0,-30 BOLD
DEFINE FONT oFont NAME "TAHOMA" SIZE 0,-15
DEFINE FONT oBold NAME "TAHOMA" SIZE 0,-15 BOLD
DEFINE DIALOG oDlg SIZE nWd, nHt PIXEL FONT oFont TRUEPIXEL ;
TITLE If( lNew, "NEW ", "EDIT " ) + "DEPOSIT" TRANSPARENT ;
BRUSH oBrush
@ 20, nWd/2-100 SAY "DEPOSIT" SIZE 200,36 PIXEL OF oDlg FONT oLarge CENTER
@ 020, nWd - 190 GET oRec:Deponum PICTURE "@!" SIZE 150,26 PIXEL OF oDlg ;
VALID ! ( Empty( oRec:Deponum ) .or. DEPOSITS->( Duplicate( oRec:Deponum, "DEPONUM", oRec:RecNo ) ) )
@ 050, nWd - 190 GET oRec:Date SIZE 150,26 PIXEL OF oDlg RIGHT ;
ACTION oRec:Date := Min( MsgDate( oRec:Date ), Date() )
@ 80-60, 40 SAY "Client:" SIZE 100,24 PIXEL OF oDlg
@ 80-60,150 GET oGetClient VAR oRec:Code SIZE 150,26 PIXEL OF oDlg ;
ACTION ( PopupBrowse( "CLIENTS", oGetClient ), ;
ReadClientInfo( oRec, oDlg ) ) ;
VALID ( ReadClientInfo( oRec, oDlg ) )
@ 125-60, 60 SAY oRec:Client SIZE 200,24 PIXEL OF oDlg FONT oBold UPDATE
@ 150-60, 60 SAY oRec:Address SIZE 200, 60 PIXEL OF oDlg UPDATE
@ 180-60,310 SAY "Text :" SIZE 100,24 PIXEL OF oDlg
@ 204-60,310 GET oRec:Details SIZE nWd-310-40,26 PIXEL OF oDlg UPDATE
@ 230-60, 40 SAY "Invoice:" SIZE 100,24 PIXEL OF oDlg
@ 254-60, 40 GET oRec:Invnum PICTURE "@!" SIZE 150,26 PIXEL OF oDlg ;
@
240-60,040 XBROWSE oBrw SIZE -40,-150+45 PIXEL OF oDlg ;
DATASOURCE aItems ;
COLUMNS 3,4,5,6,7,8 ;
HEADERS "ITEM", "DETAILS", "QTY", "UNIT","PRICE","DISCOUNT" ;
PICTURES "@!", nil, "9999.999", nil, "999.99", "999,999,999" ;
COLSIZES nil, 30 ;
CELL LINES NOBORDER FASTEDIT FOOTERS
ADD TO oBrw AT 6 HEADER "AMOUNT" DATA ROUND( oBrw:aRow[5] * oBrw:aRow[7], 0 ) ;
PICTURE "999,999,999"
ADD TO oBrw HEADER "NET" DATA ROUND( oBrw:aRow[5] * oBrw:aRow[7] - oBrw:aRow[ 8 ], 0 ) ;
PICTURE "999,999,999"
for each cCol in { "amount", "discount", "net" }
WITH OBJECT oBrw:oCol( cCol )
:nFooterType := AGGR_SUM
END
next
for each cCol in { "qty", "price", "discount" }
WITH OBJECT oBrw:oCol( cCol )
:nEditType := EDIT_GET
:bEditValid := { |o| o:VarGet() >= 0 }
:bOnChange := { || oBrw:MakeTotals( { "amount", "net" } ), oBrw:RefreshFooters(), oDlg:Update() }
END
next
for each cCol in { "details", "unit", "amount", "net" }
oBrw:oCol( cCol ):bClrStd := { || { CLR_BLACK, RGB( 240, 240, 240 ) } }
next
// AutoAppendCode
WITH OBJECT oBrw
:AddVar( "AAPPEND", nil )
:bClrStd := { || If( oBrw:aRow == oBrw:aAppend, { CLR_BLACK, CLR_YELLOW }, { CLR_BLACK, oBrw:nClrPane } ) }
:bChange := { || If( oBrw:nArrayAt < oBrw:nLen, CheckAppendRow( oBrw ), nil ) }
:bPastEof := { || If( oBrw:aAppend != nil .and. Empty( oBrw:aAppend[ 3 ] ), nil, ;
( AAdd( oBrw:aArrayData, oBrw:aAppend := AClone( aBlankItem ) ), ;
oBrw:GoBottom(), oBrw:GoLeftMost(), oBrw:RefreshCurrent(), ;
oBrw:MakeTotals(), oBrw:Refresh() ) ) }
:bKeyDown := { |k| If( k == VK_DELETE, ( oBrw:aAppend := nil, oBrw:Delete(), 0 ), nil ) }
END
WITH OBJECT oBrw:aCols[ 1 ]
:nEditType = EDIT_BUTTON
:bEditBlock = { | nRow, nCol, oCol, nKey | TableLookUp( nRow, nCol, oCol, nKey, "ITEMS" ) }
:bOnChange = { || oBrw:aAppend := nil, ReadItemInfo( oBrw:aRow, oBrw ), oBrw:RefreshCurrent(), ;
oBrw:MakeTotals(), oBrw:RefreshFooters(), oDlg:Update() }
END
WITH OBJECT oBrw
:lFlatStyle := .t.
:nStretchCol := 2
:lHScroll := .f.
:bOnRefresh := { || oDlg:Update() }
//
BrwRecSel( oBrw, "KEYNO" )
//
:MakeTotals()
:CreateFromCode()
END
@ nHt - 139 + 45, nWd - 380 SAY "TAX @" ;
SIZE 80,24 PIXEL OF oDlg RIGHT
@ nHt - 140 + 45, nWd - 280 GET oRec:TaxRate PICTURE "99.99 %" ;
SIZE 100,26 PIXEL OF oDlg RIGHT ;
VALID ( If( oRec:TaxRate >= 0, ( oDlg:Update(), .t. ), .f. ) )
@ nHT - 139 + 45, nWd - 170 SAY ;
( oRec:Tax := ROUND( oBrw:Net:nTotal * oRec:TaxRate / 100, 0 ) ) ;
PICTURE "999,999,999" SIZE 105,24 PIXEL OF oDlg UPDATE RIGHT
@ nHt - 105 + 45, nWd - 270 SAY "TOTAL" SIZE 80, 24 PIXEL OF oDlg RIGHT
@ nHt - 105 + 45, nWd - 170 SAY ;
( oRec:Total := oBrw:Net:nTotal + oRec:Tax ) ;
PICTURE "999,999,999" SIZE 105, 24 PIXEL OF oDlg UPDATE RIGHT
@ nHt - 60, 040 BTNBMP PROMPT "Save" SIZE 100,30 PIXEL OF oDlg FLAT ;
ACTION ( lSave := .t., oDlg:End() )
@ nHt - 60, 160 BTNBMP oBtn PROMPT "Cancel" SIZE 100,30 PIXEL OF oDlg FLAT ;
ACTION oDlg:End()
oBtn:lCancel := .t.
ACTIVATE DIALOG oDlg CENTERED ;
ON PAINT ( oDlg:Box( 110-60, 40, 230-60, 300 ), ;
oDlg:Line( nHt - 112 + 45, nWd - 170, nHt - 112 + 45, nWd - 55 ), ;
oDlg:Line( nHt - 78 + 45, nWd - 170, nHt - 78 + 45, nWd - 55 ), ;
oDlg:Line( nHt - 75 + 45, nWd - 170, nHt - 75 + 45, nWd - 55 ) )
if lSave
CheckAppendRow( oBrw )
if Empty( aItems ) .or. Empty( oRec:Deponum ) .or. Empty( oRec:Code ) .or. oRec:Total <= 0
else
oRec:Amount := oBrw:Net:nTotal
if ! Empty( oBrw:aDeleted )
AEval( oBrw:aDeleted, { |a| a[ 9 ] := -a[ 9 ] } )
IIT->( FW_SaveArrayToDBF( cItemFlds, oBrw:aDeleted ) )
endif
AEval( aItems, { |a| a[ 1 ] := oRec:Deponum } )
AEval( aItems, { |a,i| a[ 2 ] := i } )
IIT->( FW_SaveArrayToDBF( cItemFlds, aItems ) )
oRec:Save()
WITH OBJECT oRec:oBrw
:MakeTotals()
:RefreshFooters()
Eval( :bChange, oRec:oBrw )
END
endif
endif
RELEASE FONT oFont, oLarge
RELEASE BRUSH oBrush
return nil
```
5. **Linking Logic**: Ensure that when a deposit is created, the user can select the related invoice. This can be done via a dropdown or search functionality within the `EditDeposit` function to select an invoice number.
By implementing these changes, you will create a robust linkage between invoices and deposits, ensuring that each deposit is associated with its corresponding invoice. This setup will also allow you to easily navigate and report on the relationships between these entities.
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
... and your user manual - from the AI
# FiveWin Invoicing and Deposits Program Manual
## Table of Contents
1. Introduction
2. Installation
3. Main Window
4. Clients Management
5. Items Management
6. Invoices Management
7. Deposits Management
8. Reports
9. Closing the Program
## 1. Introduction
The FiveWin Invoicing and Deposits Program is designed to help manage client information, item details, invoices, and deposits efficiently. This manual provides a comprehensive guide on how to use the program.
## 2. Installation
To install the program, follow these steps:
1. Ensure you have the FiveWin libraries and a compatible Harbour compiler installed.
2. Place the program files in a directory.
3. Compile the program using the Harbour compiler.
4. Run the executable file generated.
## 3. Main Window
Upon starting the program, the main window will appear with the following features:
- **Menu Bar**: Provides access to various functionalities such as managing clients, items, invoices, and deposits.
- **Button Bar**: Contains buttons for quick access to invoices, deposits, clients, items, and exiting the program.
- **Status Bar**: Displays the current status and other information.
## 4. Clients Management
To manage clients, follow these steps:
1. Click on the **Clients** button in the Button Bar or select **Clients** from the Menu Bar.
2. The Clients window will open, displaying a list of clients.
3. Use the buttons to add, edit, or delete clients.
- **Add**: Opens a form to enter new client details.
- **Edit**: Opens a form to edit the selected client's details.
- **Delete**: Removes the selected client from the database.
4. You can also export client data to CSV or print a report from this window.
## 5. Items Management
To manage items, follow these steps:
1. Click on the **Items** button in the Button Bar or select **Items** from the Menu Bar.
2. The Items window will open, displaying a list of items.
3. Use the buttons to add, edit, or delete items.
- **Add**: Opens a form to enter new item details.
- **Edit**: Opens a form to edit the selected item's details.
- **Delete**: Removes the selected item from the database.
4. You can print an item report from this window.
## 6. Invoices Management
To manage invoices, follow these steps:
1. Click on the **Invoices** button in the Button Bar or select **Invoices** from the Menu Bar.
2. The Invoices window will open, displaying a list of invoices.
3. Use the buttons to add, edit, or delete invoices.
- **Add**: Opens a form to create a new invoice.
- **Edit**: Opens a form to edit the selected invoice's details.
- **Delete**: Removes the selected invoice from the database.
4. You can view, print, or generate an HTML report of the invoice from this window.
## 7. Deposits Management
To manage deposits, follow these steps:
1. Click on the **Deposits** button in the Button Bar or select **Deposits** from the Menu Bar.
2. The Deposits window will open, displaying a list of deposits.
3. Use the buttons to add, edit, or delete deposits.
- **Add**: Opens a form to create a new deposit.
- **Edit**: Opens a form to edit the selected deposit's details.
- **Delete**: Removes the selected deposit from the database.
4. You can view, print, or generate an HTML report of the deposit from this window.
## 8. Reports
To generate reports, follow these steps:
1. Navigate to the desired section (Clients, Items, Invoices, Deposits).
2. Use the **Print** or **HTML Report** buttons to generate a report.
3. Follow the prompts to customize and print the report.
## 9. Closing the Program
To close the program, follow these steps:
1. Click on the **Exit** button in the Button Bar or select **Exit** from the Menu Bar.
2. The program will close, ensuring all data is saved and properly exited.
---
This manual should help you understand and use the main features of the program. Adjustments can be made based on specific functionalities and additional details provided in your actual implementation.
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
... and the flowchart for the programm - developement
Hello friends,
I believe that a year ago, we could not have imagined how much AI would assist in programming.
Best regards,
Otto
https://mybergland.com/flowchartyunus.png
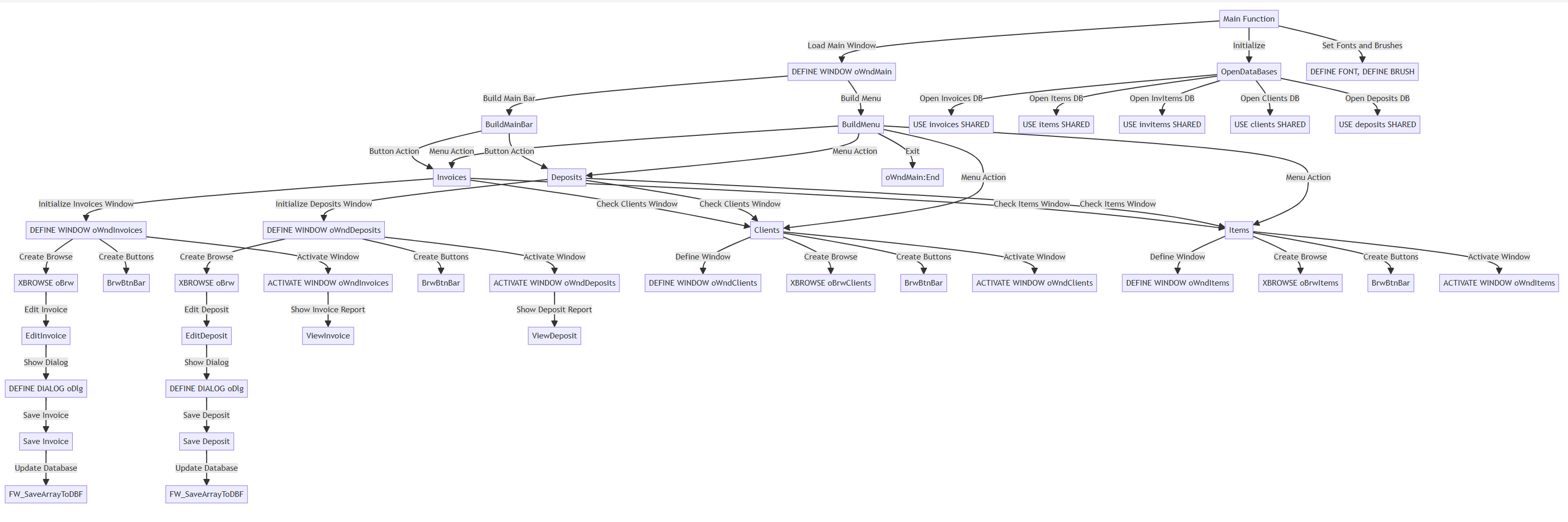
Hello friends,
I believe that a year ago, we could not have imagined how much AI would assist in programming.
Best regards,
Otto
https://mybergland.com/flowchartyunus.png
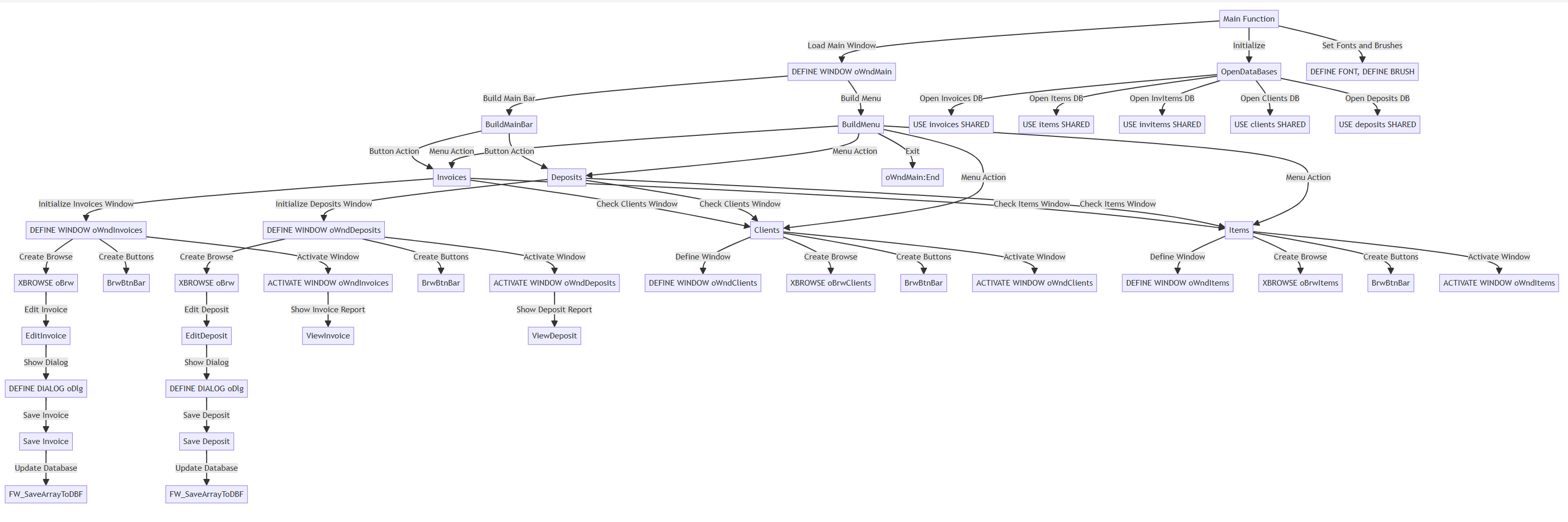
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
- Enrico Maria Giordano
- Posts: 8753
- Joined: Thu Oct 06, 2005 8:17 pm
- Location: Roma - Italia
- Has thanked: 1 time
- Been thanked: 4 times
- Contact:
Re: Need Help giide for my program
That's a very bad way of programming to me.Otto wrote:start with a ChatGPT prompt.
- Carles
- Posts: 1149
- Joined: Fri Feb 10, 2006 2:34 pm
- Location: Barcelona
- Been thanked: 7 times
- Contact:
Re: Need Help giide for my program
Enrico Maria Giordano wrote: That's a very bad way of programming to me.
+1
For me too, it's like starting to be a doctor without having knowledge...

Nice weekend.
C.
Salutacions, saludos, regards
"...programar es fácil, hacer programas es difícil..."
UT Page -> https://carles9000.github.io/
Forum UT -> https://discord.gg/bq8a9yGMWh
Skype -> https://join.skype.com/cnzQg3Kr1dnk
"...programar es fácil, hacer programas es difícil..."
UT Page -> https://carles9000.github.io/
Forum UT -> https://discord.gg/bq8a9yGMWh
Skype -> https://join.skype.com/cnzQg3Kr1dnk
- karinha
- Posts: 7940
- Joined: Tue Dec 20, 2005 7:36 pm
- Location: São Paulo - Brasil
- Been thanked: 3 times
- Contact:
Re: Need Help giide for my program
+ 1Enrico Maria Giordano wrote:That's a very bad way of programming to me.Otto wrote:start with a ChatGPT prompt.
Master Otto was unhappy in his response.
Regards, saludos.
João Santos - São Paulo - Brasil - Phone: +55(11)95150-7341
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
Hello friends,
To AI support
Way back when I was a little boy, the industry just started selling electric stoves to households.
I still remember the conversations about how housewives claimed that you couldn't really cook with electric stoves.
But today, it's standard. I have truly witnessed this change. At first, when kitchens were converted to electric stoves, a small wood stove was added to dispel any concerns.
However, when Yunus comes back after a break, I think it's good for him to see what is possible today.
You don't have to use AI, but ignoring it is also not right because it is a reality.
And just by the way, the proposed solution path leads to the result.
Of course, the first PROMPT does not immediately provide a workable solution.
But a few months ago, there were no hits for FIVEWIN at all. Now, HARBOUR and FIVEWIN are already well supported by AI.
And we can really be glad about that, otherwise we could say goodbye to the market right away.
In the future, it will be impossible to keep up with the competition without AI.
Best regards,
Otto
To AI support
Way back when I was a little boy, the industry just started selling electric stoves to households.
I still remember the conversations about how housewives claimed that you couldn't really cook with electric stoves.
But today, it's standard. I have truly witnessed this change. At first, when kitchens were converted to electric stoves, a small wood stove was added to dispel any concerns.
However, when Yunus comes back after a break, I think it's good for him to see what is possible today.
You don't have to use AI, but ignoring it is also not right because it is a reality.
And just by the way, the proposed solution path leads to the result.
Of course, the first PROMPT does not immediately provide a workable solution.
But a few months ago, there were no hits for FIVEWIN at all. Now, HARBOUR and FIVEWIN are already well supported by AI.
And we can really be glad about that, otherwise we could say goodbye to the market right away.
In the future, it will be impossible to keep up with the competition without AI.
Best regards,
Otto
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
- Antonio Linares
- Site Admin
- Posts: 42529
- Joined: Thu Oct 06, 2005 5:47 pm
- Location: Spain
- Has thanked: 31 times
- Been thanked: 77 times
- Contact:
Re: Need Help giide for my program
+1
Otto wrote:Hello friends,
To AI support
Way back when I was a little boy, the industry just started selling electric stoves to households.
I still remember the conversations about how housewives claimed that you couldn't really cook with electric stoves.
But today, it's standard. I have truly witnessed this change. At first, when kitchens were converted to electric stoves, a small wood stove was added to dispel any concerns.
However, when Yunus comes back after a break, I think it's good for him to see what is possible today.
You don't have to use AI, but ignoring it is also not right because it is a reality.
And just by the way, the proposed solution path leads to the result.
Of course, the first PROMPT does not immediately provide a workable solution.
But a few months ago, there were no hits for FIVEWIN at all. Now, HARBOUR and FIVEWIN are already well supported by AI.
And we can really be glad about that, otherwise we could say goodbye to the market right away.
In the future, it will be impossible to keep up with the competition without AI.
Best regards,
Otto
- Enrico Maria Giordano
- Posts: 8753
- Joined: Thu Oct 06, 2005 8:17 pm
- Location: Roma - Italia
- Has thanked: 1 time
- Been thanked: 4 times
- Contact:
Re: Need Help giide for my program
I use it every day but the answers are always useless. I hope it will improve in the future.Otto wrote:You don't have to use AI, but ignoring it is also not right because it is a reality.
-
- Posts: 85
- Joined: Wed Nov 19, 2014 1:04 pm
- Contact:
Re: Need Help giide for my program
+1
Hello friends,
To AI support
Way back when I was a little boy, the industry just started selling electric stoves to households.
I still remember the conversations about how housewives claimed that you couldn't really cook with electric stoves.
But today, it's standard. I have truly witnessed this change. At first, when kitchens were converted to electric stoves, a small wood stove was added to dispel any concerns.
However, when Yunus comes back after a break, I think it's good for him to see what is possible today.
You don't have to use AI, but ignoring it is also not right because it is a reality.
And just by the way, the proposed solution path leads to the result.
Of course, the first PROMPT does not immediately provide a workable solution.
But a few months ago, there were no hits for FIVEWIN at all. Now, HARBOUR and FIVEWIN are already well supported by AI.
And we can really be glad about that, otherwise we could say goodbye to the market right away.
In the future, it will be impossible to keep up with the competition without AI.
Best regards,
Otto
Regards
Yunus
FWH 21.02
Yunus
FWH 21.02
- Otto
- Posts: 6404
- Joined: Fri Oct 07, 2005 7:07 pm
- Has thanked: 24 times
- Been thanked: 2 times
- Contact:
Re: Need Help giide for my program
Hallo Endico,
>I use it every day but the answers are always useless. I hope it will improve in the future.
https://mybergland.com/fwforum/fivewin.html
Best regards,
Otto
>I use it every day but the answers are always useless. I hope it will improve in the future.
Please take a look at the answer.I asked this question, for example:
A program is created by us as application programmers, for example, as follows:
#include "fivewin.ch"
function main
return
How is FiveWin loaded or what is that called?
https://mybergland.com/fwforum/fivewin.html
Best regards,
Otto
Last edited by Otto on Sat Jul 20, 2024 9:54 am, edited 1 time in total.
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************
mod harbour - Vamos a la conquista de la Web
modharbour.org
https://www.facebook.com/groups/modharbour.club
********************************************************************