Saludos fivewiners
Al Grano.
Recibo informacion de 3 turnos por dia en una empresa, para 4 procesos productivos
-------------1 Turno ----- 2 Turno ------- 3 Turno
Cepillado---- 25 ------------ 36 ------------ 30
Corte------- 20 ------------ 32 ------------ 28
Ensamble--- 18 ------------ 28 ------------ 27
Despacho-- 16 ------------ 24 ------------ 25
Todo en metros cubicos. Un informe diario. Debo comparar los dias en cuanto a turno y proceso, todos los informes del mes en algun momento los tengo en arreglos.
Hay alguna forma de comparar estos arreglos entre dias del mes.
Que dias fueron muy iguales, cuales muy distintos, que mezcla de turno y proceso vario mas.
Algo asi como EQUALS() de java
Cualquier ayuda sera bienvenida, podria darme el trabajo de iteracion repetitiva, pero si alguien tiene alguna idea distinta la considerare.
Desde Chile,
Adolfo
Comparar matrices, arreglos
Comparar matrices, arreglos

http://www.xdata.cl - Desarrollo Inteligente
----------
Asus TUF F15, 32GB Ram, 2 * 1 TB NVME M.2, GTX 1650
- Antonio Linares
- Site Admin
- Posts: 42521
- Joined: Thu Oct 06, 2005 5:47 pm
- Location: Spain
- Has thanked: 31 times
- Been thanked: 76 times
- Contact:
Re: Comparar matrices, arreglos
Estimado Adolfo,
Le he preguntado a Claude ya que lo idóneo es comenzar con el análisis de la IA:
Le he preguntado a Claude ya que lo idóneo es comenzar con el análisis de la IA:
Code: Select all | Expand
#include "hbclass.ch"
CREATE CLASS InformeProduccion
VAR fecha
VAR datos
METHOD New(cFecha, aDatos)
METHOD Equals(oOtro)
METHOD Diferencia(oOtro)
ENDCLASS
METHOD New(cFecha, aDatos) CLASS InformeProduccion
::fecha := cFecha
::datos := aDatos
RETURN Self
METHOD Equals(oOtro) CLASS InformeProduccion
LOCAL nI, nJ
FOR nI := 1 TO Len(::datos)
FOR nJ := 1 TO Len(::datos[nI])
IF ::datos[nI][nJ] != oOtro:datos[nI][nJ]
RETURN .F.
ENDIF
NEXT
NEXT
RETURN .T.
METHOD Diferencia(oOtro) CLASS InformeProduccion
LOCAL nDif := 0, nI, nJ
FOR nI := 1 TO Len(::datos)
FOR nJ := 1 TO Len(::datos[nI])
nDif += (::datos[nI][nJ] - oOtro:datos[nI][nJ]) ^ 2
NEXT
NEXT
RETURN SQRT(nDif)
FUNCTION CompararInformes(aInformes)
LOCAL aSimilitudes := {}, aDiferencias := {}
LOCAL nI, nJ, oInf1, oInf2, nDif
FOR nI := 1 TO Len(aInformes) - 1
oInf1 := aInformes[nI]
FOR nJ := nI + 1 TO Len(aInformes)
oInf2 := aInformes[nJ]
IF oInf1:Equals(oInf2)
AAdd(aSimilitudes, {oInf1:fecha, oInf2:fecha})
ELSE
nDif := oInf1:Diferencia(oInf2)
AAdd(aDiferencias, {oInf1:fecha, oInf2:fecha, nDif})
ENDIF
NEXT
NEXT
RETURN {aSimilitudes, aDiferencias}
FUNCTION AnalizarVariacion(aInformes)
LOCAL aVariacion := {}, nMaxVar := 0
LOCAL nProceso, nTurno, nI, nJ, nK, nSum, nMedia, nVar
LOCAL nMaxProceso := 0, nMaxTurno := 0
// Inicializar array de variación
FOR nI := 1 TO 4
AAdd(aVariacion, Array(3))
AFill(aVariacion[nI], 0)
NEXT
// Calcular variación para cada celda
FOR nProceso := 1 TO 4
FOR nTurno := 1 TO 3
nSum := 0
FOR nK := 1 TO Len(aInformes)
nSum += aInformes[nK]:datos[nProceso][nTurno]
NEXT
nMedia := nSum / Len(aInformes)
nVar := 0
FOR nK := 1 TO Len(aInformes)
nVar += (aInformes[nK]:datos[nProceso][nTurno] - nMedia) ^ 2
NEXT
nVar := nVar / Len(aInformes)
aVariacion[nProceso][nTurno] := SQRT(nVar)
IF aVariacion[nProceso][nTurno] > nMaxVar
nMaxVar := aVariacion[nProceso][nTurno]
nMaxProceso := nProceso
nMaxTurno := nTurno
ENDIF
NEXT
NEXT
RETURN {nMaxProceso, nMaxTurno}
PROCEDURE Main()
LOCAL aInformes := {}
LOCAL aSimilitudes, aDiferencias, aMaxVar
// Crear informes de ejemplo
AAdd(aInformes, InformeProduccion():New("2024-08-01", ;
{{25, 36, 30}, ;
{20, 32, 28}, ;
{18, 28, 27}, ;
{16, 24, 25}}))
AAdd(aInformes, InformeProduccion():New("2024-08-02", ;
{{24, 35, 31}, ;
{21, 33, 27}, ;
{19, 29, 26}, ;
{17, 25, 24}}))
// Comparar informes
aSimilitudes := CompararInformes(aInformes)[1]
aDiferencias := CompararInformes(aInformes)[2]
// Mostrar resultados
? "Informes idénticos:"
AEval(aSimilitudes, {|x| QOut(x[1] + " y " + x[2])})
? "Diferencias entre informes:"
AEval(aDiferencias, {|x| QOut(x[1] + " y " + x[2] + ": " + AllTrim(Str(x[3])))})
// Analizar variación
aMaxVar := AnalizarVariacion(aInformes)
? "La mayor variación ocurrió en el proceso " + AllTrim(Str(aMaxVar[1])) + ;
" y turno " + AllTrim(Str(aMaxVar[2]))
RETURN
Definimos una clase InformeProduccion que representa un informe diario de producción.
Implementamos métodos Equals() y Diferencia() en la clase para comparar informes.
La función CompararInformes() compara todos los pares de informes, identificando los idénticos y calculando las diferencias.
La función AnalizarVariacion() encuentra qué combinación de proceso y turno varió más a lo largo del período.
En la función Main(), creamos algunos informes de ejemplo y mostramos cómo usar las funciones de comparación y análisis.
- Antonio Linares
- Site Admin
- Posts: 42521
- Joined: Thu Oct 06, 2005 5:47 pm
- Location: Spain
- Has thanked: 31 times
- Been thanked: 76 times
- Contact:
Re: Comparar matrices, arreglos
También le he preguntado acerca de cual sería la forma de compararlos visualmente y directamente ha construido esto:
Puedes pedirle el código en HTML con lo que lo podrías visualizar usando la clase TWebView2
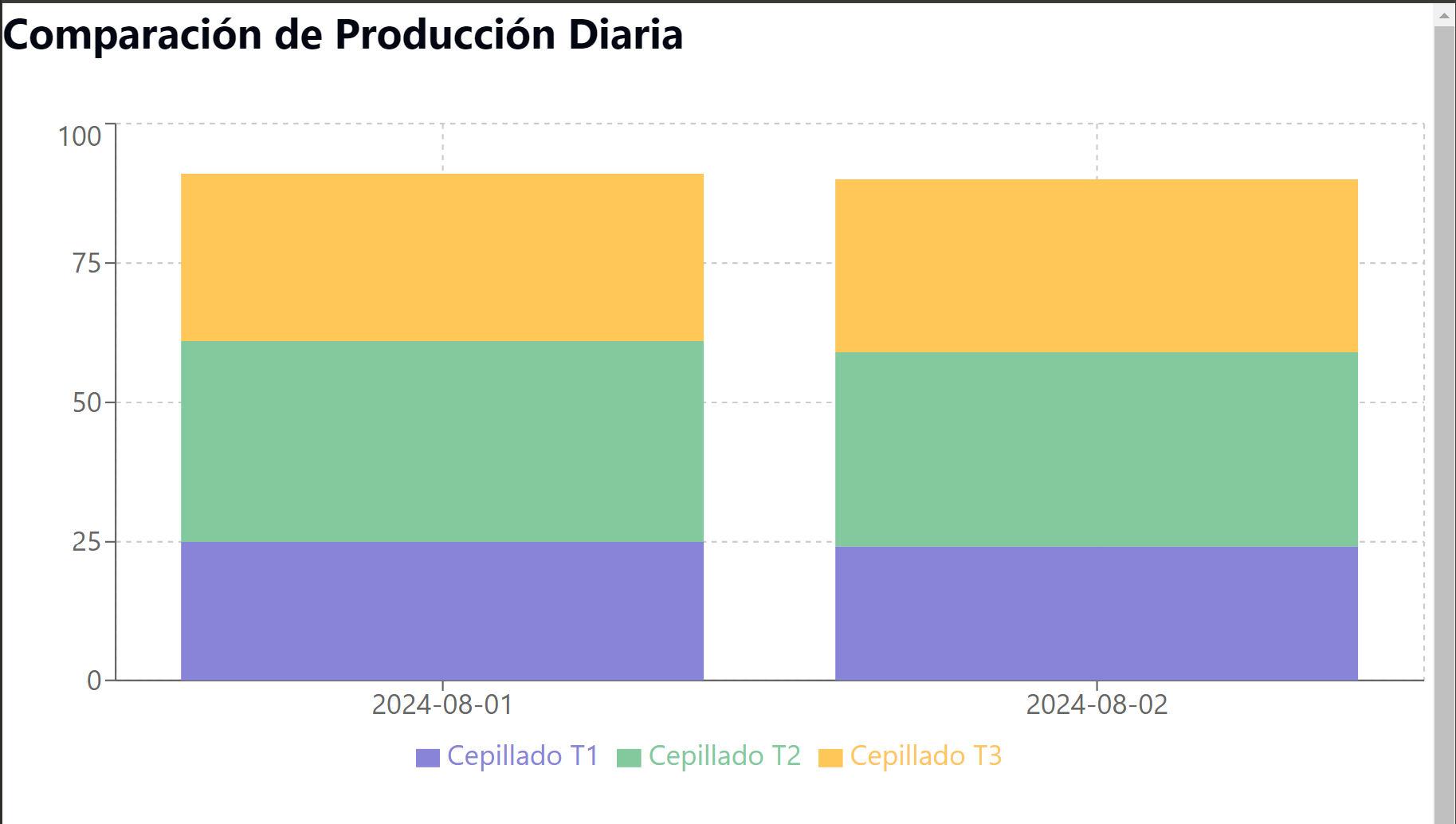
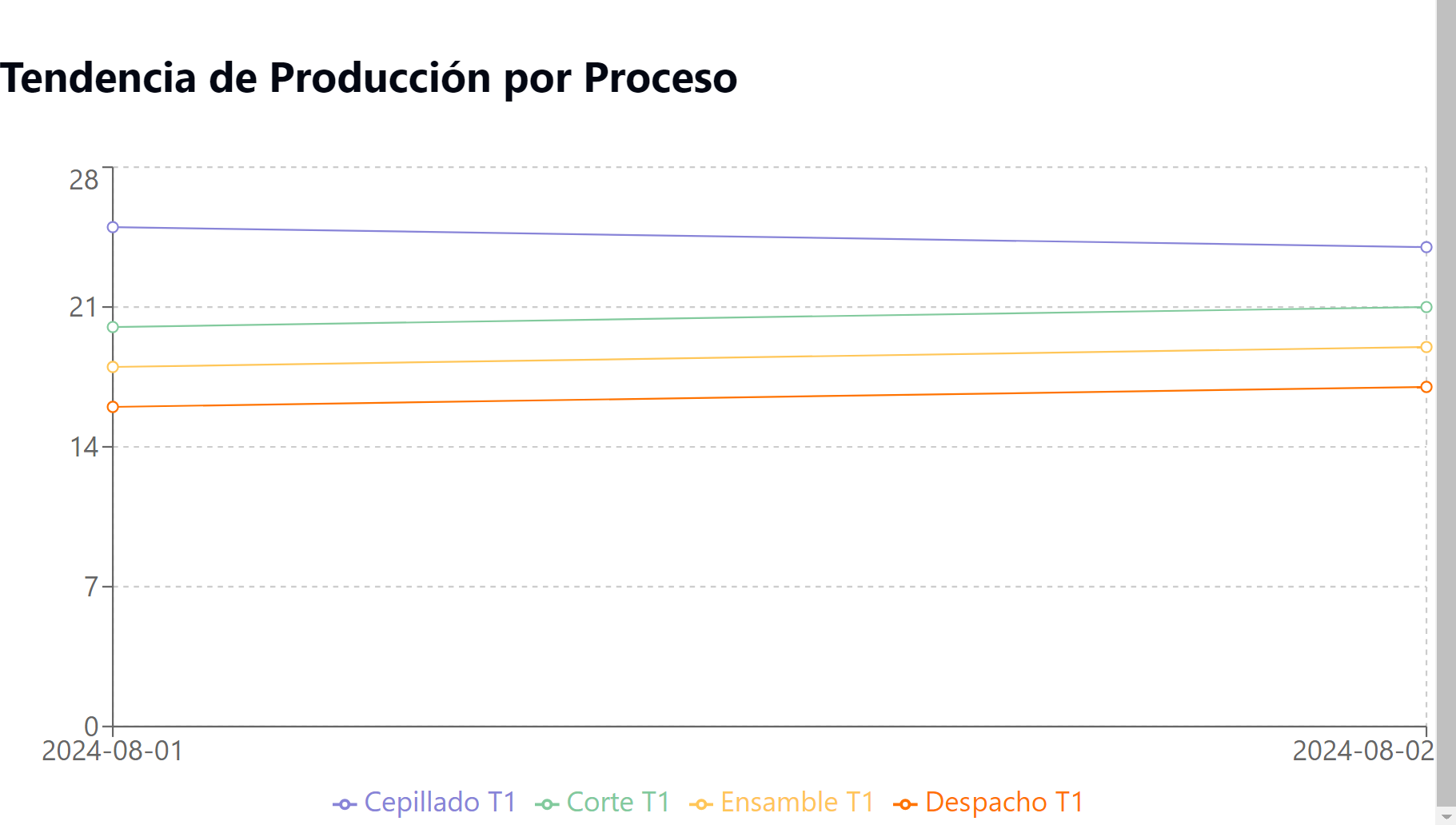
Puedes pedirle el código en HTML con lo que lo podrías visualizar usando la clase TWebView2
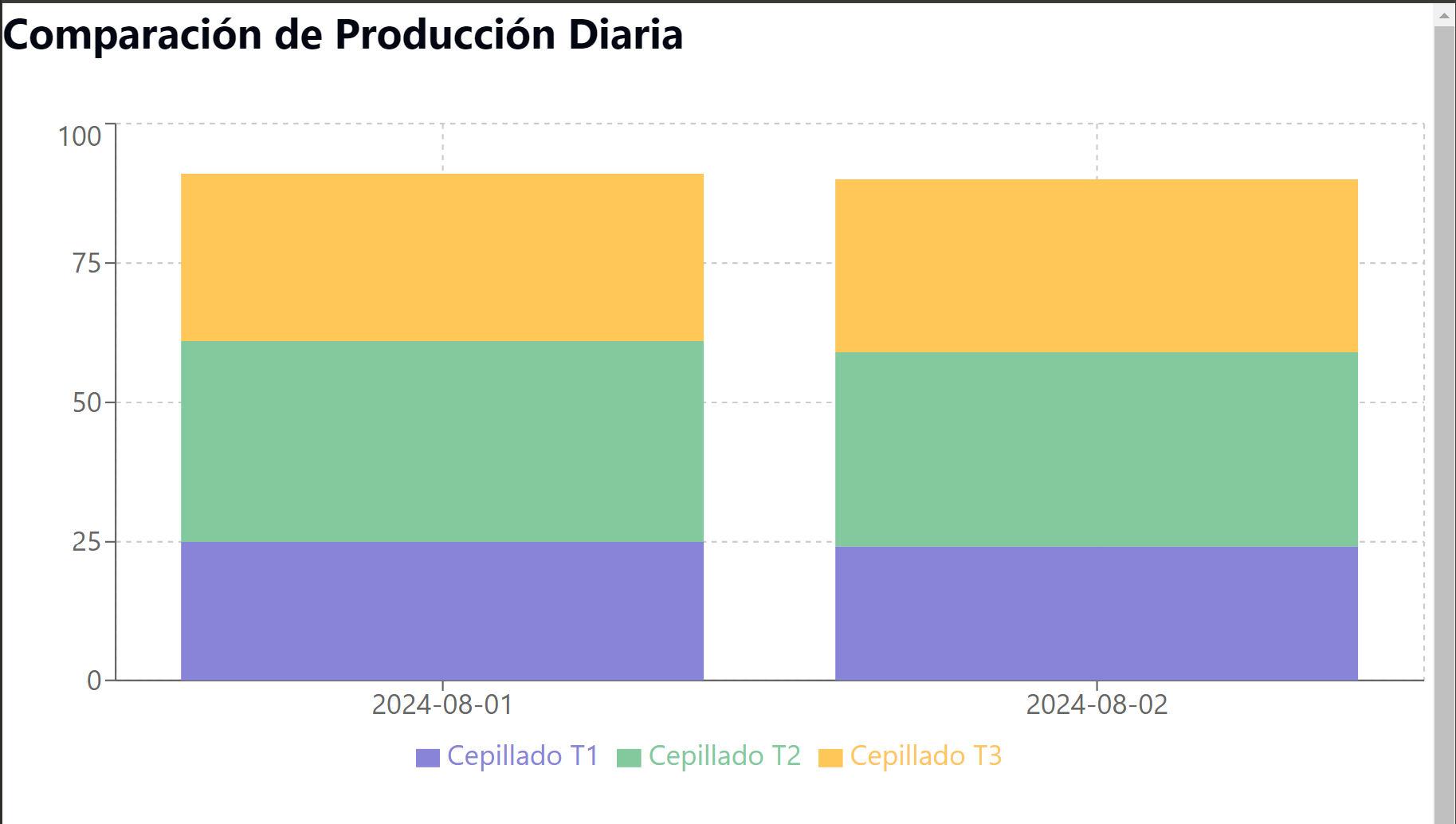
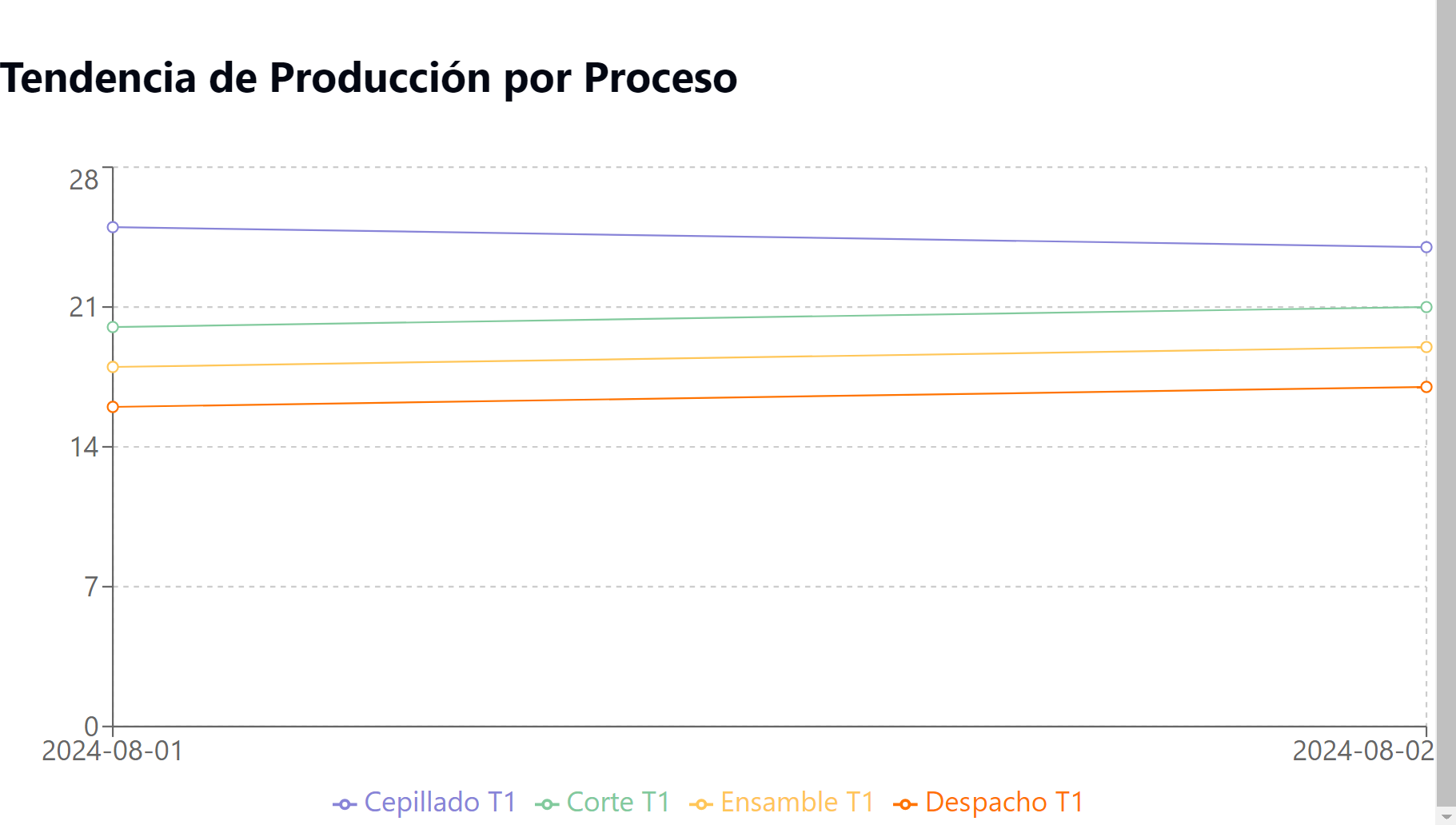
Re: Comparar matrices, arreglos
Muchisimas gracias Antonio
Le meto prueba y codigo y te comento como me va
Saludos desde Chile
Le meto prueba y codigo y te comento como me va
Saludos desde Chile

http://www.xdata.cl - Desarrollo Inteligente
----------
Asus TUF F15, 32GB Ram, 2 * 1 TB NVME M.2, GTX 1650